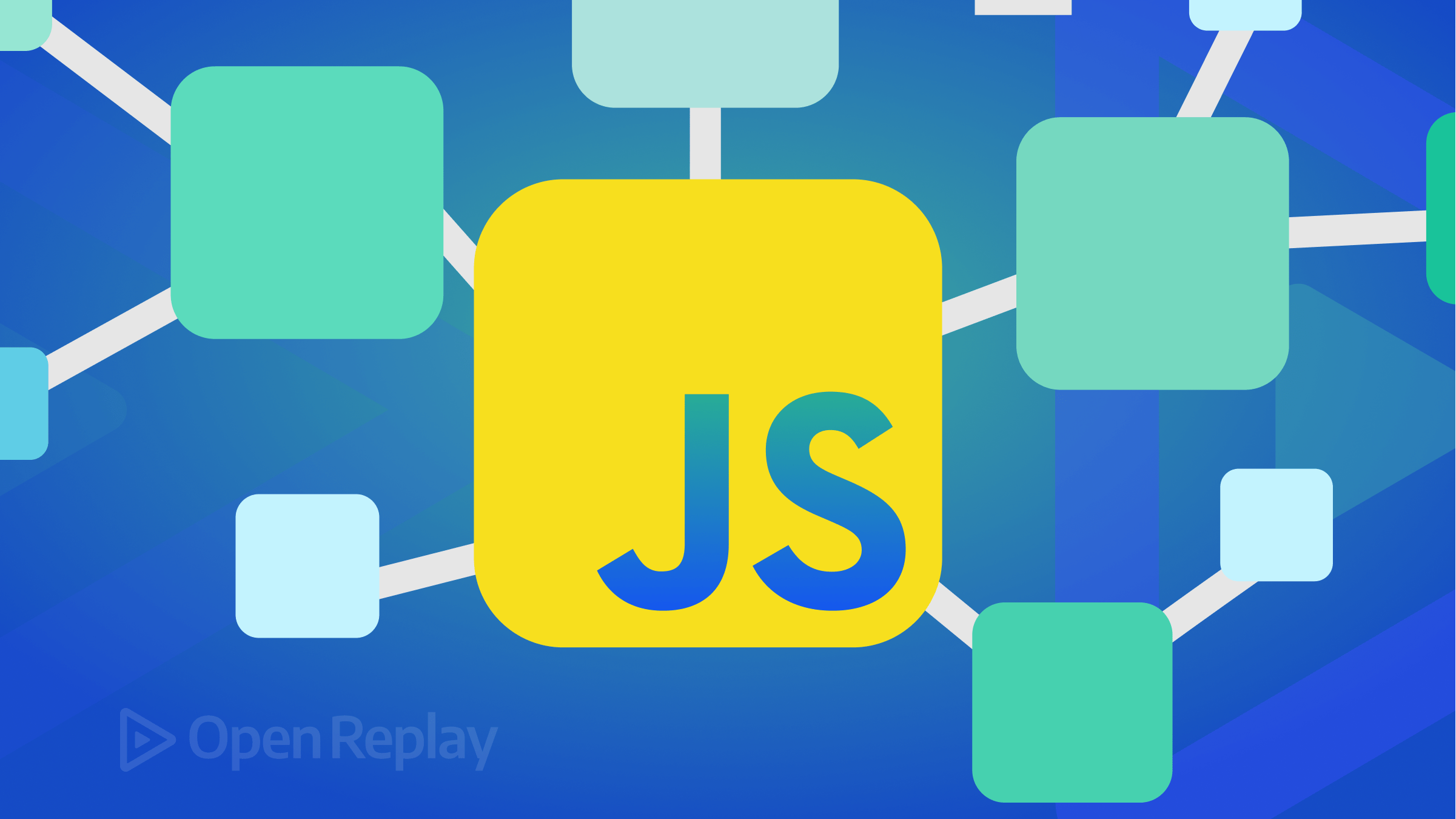
JavaScript is a versatile and powerful programming language used to add interactive elements and functionality to websites. One of the fundamental and unique features of JavaScript is its ability to perform operations asynchronously. This means JavaScript can execute multiple operations at once, which helps in improving the performance and responsiveness of web applications.
Understanding asynchronous JavaScript is crucial for developers, especially when dealing with tasks that might take a while to complete, such as fetching data from a server, reading files, or handling user interactions. This blog will guide you through the basics of asynchronous programming in JavaScript, its importance, and how you can handle asynchronous operations.
Understanding Synchronous and Asynchronous Programming
Before diving into asynchronous JavaScript, let's first understand the difference between synchronous and asynchronous programming.
Synchronous Programming
In synchronous programming, tasks are executed in a sequential order. Each operation must complete before the next one starts. This can lead to delays and unresponsive applications if one of the operations takes a long time to complete.
Example:
console.log("Start");
function fetchData() {
// Simulate a network request
let startTime = new Date().getTime();
while (new Date().getTime() < startTime + 3000); // 3 seconds delay
console.log("Data fetched");
}
fetchData();
console.log("End");
In the above code, the output will be:
Start
Data fetched
End
Here, the fetchData
function blocks the execution until it completes, which takes 3 seconds. This means that the console.log("End")
statement will not execute until fetchData
is finished.
Asynchronous Programming
Asynchronous programming allows tasks to be executed in parallel. Operations do not wait for each other to complete. This makes it possible to perform time-consuming operations without blocking the main thread, thus keeping the application responsive.
Example:
console.log("Start");
function fetchData() {
setTimeout(() => {
console.log("Data fetched");
}, 3000); // 3 seconds delay
}
fetchData();
console.log("End");
In this example, the output will be:
Start
End
Data fetched
Here, the setTimeout
function schedules the callback to be executed after 3 seconds, but it does not block the execution of subsequent code. Therefore, console.log("End")
executes immediately after fetchData()
, and console.log("Data fetched")
runs after 3 seconds.
Importance of Asynchronous JavaScript
- Improved Performance: By not blocking the main thread, asynchronous JavaScript allows other operations to run simultaneously, leading to improved performance and faster execution times.
- Responsive User Interfaces: User interfaces remain responsive even when handling long-running tasks, as the UI thread is not blocked by these operations.
- Better Resource Utilization: Asynchronous operations make better use of system resources like the CPU and network, as they can perform other tasks while waiting for operations to complete.
- Handling I/O Operations: Asynchronous programming is ideal for handling input/output (I/O) operations such as network requests, file operations, and database queries, which can take a considerable amount of time.
Asynchronous Programming in JavaScript
JavaScript, by default, is a single-threaded language but uses an event-driven architecture to handle asynchronous operations. Here's how JavaScript manages asynchronous tasks:
Event Loop
The event loop is a fundamental concept in asynchronous JavaScript. It continuously checks the call stack and the task queue to ensure that asynchronous operations are handled properly.
Here's a simplified explanation of how the event loop works:
- Call Stack: This is where JavaScript executes code. It follows the Last In, First Out (LIFO) principle.
- Task Queue: This holds asynchronous tasks that are waiting to be executed. These tasks are moved from the task queue to the call stack when it is free.
Web APIs
Web APIs, provided by the browser, are used to handle asynchronous operations such as timers (setTimeout
, setInterval
), AJAX requests, and events.
Example of Web API usage:
console.log("Start");
setTimeout(() => {
console.log("Data fetched");
}, 3000);
console.log("End");
In this example, setTimeout
is a Web API that schedules the callback to run after 3 seconds. The callback is not executed immediately; instead, it is moved to the task queue and waits for the call stack to be free.
Callbacks
Callbacks are functions that are passed as arguments to other functions and are executed after a certain operation is completed. Callbacks are the traditional way to handle asynchronous operations in JavaScript.
Example of using callbacks:
console.log("Start");
function fetchData(callback) {
setTimeout(() => {
console.log("Data fetched");
callback();
}, 3000);
}
fetchData(() => {
console.log("Callback executed");
});
console.log("End");
Here, the fetchData
function takes a callback function as an argument. Once the data fetching is complete, the callback function is executed.
Promises
Promises are a more modern and cleaner way to handle asynchronous operations compared to callbacks. A promise represents a value that may be available now, in the future, or never.
Example of using Promises:
console.log("Start");
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Data fetched");
resolve();
}, 3000);
});
}
fetchData().then(() => {
console.log("Promise resolved");
});
console.log("End");
In this example, fetchData
returns a promise that resolves after 3 seconds. The then
method is used to specify what should happen once the promise is resolved.
Async/Await
async/await
is the latest syntax introduced in ES2017 for handling asynchronous operations. It makes asynchronous code look synchronous and is built on top of promises.
Example of using Async/Await:
console.log("Start");
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Data fetched");
resolve();
}, 3000);
});
}
async function executeFetchData() {
await fetchData();
console.log("Data processed");
}
executeFetchData();
console.log("End");
Here, the fetchData
function returns a promise, and executeFetchData
is an async
function that uses the await
keyword to wait for the promise to resolve. This makes the code easier to read and maintain.
Handling Asynchronous Operations
Handling asynchronous operations is crucial for building efficient and responsive web applications. Let's explore different methods to handle these operations effectively.
Callbacks
Callbacks are functions that are executed after a specific event or operation is completed. They are the traditional way to handle asynchronous operations in JavaScript.
Example of using callbacks:
console.log("Start");
function fetchData(callback) {
setTimeout(() => {
console.log("Data fetched");
callback();
}, 3000);
}
fetchData(() => {
console.log("Callback executed");
});
console.log("End");
Advantages:
- Simple to use for simple asynchronous operations.
Disadvantages:
- Can lead to callback hell with multiple nested callbacks.
Promises
Promises are a more modern way to handle asynchronous operations. They represent a value that will be available in the future and can be handled in a more structured way.
Example of using Promises:
console.log("Start");
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Data fetched");
resolve();
}, 3000);
});
}
fetchData().then(() => {
console.log("Promise resolved");
}).catch((error) => {
console.error("Error:", error);
});
console.log("End");
Advantages:
- Avoids callback hell.
- Provides a more readable and maintainable code structure.
Disadvantages:
- Can be complex for beginners to understand.
Async/Await
async/await
is the latest syntax introduced for handling asynchronous operations in JavaScript. It makes the code look synchronous and easier to read and maintain.
Example of using Async/Await:
console.log("Start");
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log("Data fetched");
resolve();
}, 3000);
});
}
async function executeFetchData() {
try {
await fetchData();
console.log("Data processed");
} catch (error) {
console.error("Error:", error);
}
}
executeFetchData();
console.log("End");
Advantages:
- Makes asynchronous code look synchronous.
- Easier to read and maintain.
- Better error handling with
try/catch
.
Disadvantages:
- Requires understanding of promises.
Common Asynchronous Operations in JavaScript
JavaScript handles various types of asynchronous operations, such as:
- Timers: Scheduled using
setTimeout
andsetInterval
functions. - Network Requests: Made using
XMLHttpRequest
or the more modernfetch
API. - File Operations: Used in environments like Node.js.
- User Input: Handling events like clicks, key presses, etc.
Timers
Timers are used to schedule functions to be executed after a specified delay or repeatedly at fixed intervals.
setTimeout
setTimeout
is used to schedule a function to be executed after a specified delay.
Syntax:
setTimeout(function, delay);
Example:
console.log("Start");
setTimeout(() => {
console.log("This will run after 2 seconds");
}, 2000);
console.log("End");
setInterval
setInterval
is used to schedule a function to be executed repeatedly at fixed intervals.
Syntax:
setInterval(function, interval);
Example:
let count = 0;
const intervalId = setInterval(() => {
count++;
console.log("Count is:", count);
if (count === 5) {
clearInterval(intervalId);
console.log("Interval cleared");
}
}, 1000);
Network Requests
Network requests are a common example of asynchronous operations. JavaScript provides several ways to make network requests, including XMLHttpRequest
and the fetch
API.
XMLHttpRequest
XMLHttpRequest
is an older method to make HTTP requests. It is less common in modern JavaScript development.
Example:
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log("Data received:", xhr.responseText);
}
};
xhr.open("GET", "https://api.example.com/data", true);
xhr.send();
Fetch API
The Fetch API is a modern way to make HTTP requests. It returns a promise that resolves to the Response
object representing the response to the request.
Example:
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error:", error));
File Operations
File operations are another example of asynchronous operations, commonly used in server-side environments like Node.js.
Example (Node.js):
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err);
return;
}
console.log("File content:", data);
});
console.log("File read started");
User Input
Handling user input involves asynchronous operations, such as event listeners for button clicks or key presses.
Example:
document.getElementById("myButton").addEventListener("click", () => {
console.log("Button clicked");
});
console.log("Event listener added");
Best Practices for Asynchronous JavaScript
Asynchronous JavaScript can be tricky to get right, but following best practices can help you avoid common pitfalls and write cleaner code.
- Use Promises or Async/Await: Avoid using callbacks as they can lead to callback hell.
- Handle Errors: Always handle errors in asynchronous operations using
.catch
for promises andtry/catch
blocks for async/await. - Keep Code Readable: Write clean and readable code using modern features like async/await.
- Avoid Blocking Operations: Avoid long-running synchronous operations that can block the main thread.
Common Error Handling Patterns
Proper error handling is essential in asynchronous JavaScript to handle errors gracefully and improve the robustness of your applications.
Handling Errors with Promises
Promises provide a .catch
method to handle errors that occur during asynchronous operations.
Example:
fetch("https://api.example.com/data")
.then(response => response.json())
.then(data => console.log("Data received:", data))
.catch(error => console.error("Error:", error));
Handling Errors with Async/Await
With async/await, you can use try/catch
blocks to handle errors in a synchronous-like manner.
Example:
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log("Data received:", data);
} catch (error) {
console.error("Error:", error);
}
}
fetchData();
Real-world Applications of Asynchronous JavaScript
Asynchronous JavaScript has numerous applications in real-world scenarios, including:
- Fetching Data from APIs: Fetching data from servers without blocking the main thread.
- File Reading and Writing: Handling file operations without freezing the application.
- Handling User Events: Responding to user interactions smoothly.
- Animating Web Pages: Performing animations without blocking the user interface.
Challenges in Asynchronous JavaScript
While asynchronous programming in JavaScript offers many benefits, it also comes with its own set of challenges.
- Complexity: Asynchronous code can be more complex to write, read, and debug compared to synchronous code.
- Concurrency Issues: Handling multiple asynchronous operations can lead to race conditions and other concurrency issues.
- Error Handling: Proper error handling is crucial to avoid unhandled promise rejections and other errors.
Conclusion
Asynchronous JavaScript is a powerful feature that allows developers to build efficient and responsive web applications. By understanding the basics of asynchronous programming, you can write cleaner, more maintainable code that handles asynchronous operations effectively.
In this blog, we have covered:
- The difference between synchronous and asynchronous programming.
- The event loop and Web APIs.
- Different methods to handle asynchronous operations, including callbacks, promises, and async/await.
- Common error handling patterns.
- Real-world applications and challenges of asynchronous programming.
By following best practices and understanding the fundamentals, you can effectively use asynchronous JavaScript in your projects.
Additional Resources
- MDN Web Docs - Asynchronous JavaScript
- JavaScript.info - Asynchronous JavaScript
- You Don't Know JS: Async & Performance
Check out these resources for more in-depth knowledge and advanced topics in asynchronous JavaScript.
Q&A
Feel free to ask any questions or provide feedback. Here are some common questions related to asynchronous JavaScript:
-
Q: What is the event loop in JavaScript?
- A: The event loop is a mechanism that continuously monitors the call stack and task queue to handle asynchronous operations.
-
Q: Can I convert callbacks to promises?
- A: Yes, you can convert callbacks to promises using the
Promise
constructor.
- A: Yes, you can convert callbacks to promises using the
-
Q: How do async/await differ from promises?
- A: Async/await is syntax sugar over promises that makes asynchronous code look more synchronous and easier to read.
-
Q: What is the difference between
setTimeout
andsetInterval
?- A:
setTimeout
schedules a function to be executed after a delay, whilesetInterval
repeatedly executes a function at fixed intervals.
- A:
-
Q: How can I handle multiple asynchronous operations simultaneously?
- A: You can handle multiple asynchronous operations using
Promise.all
,Promise.race
, orasync/await
withPromise.all
.
- A: You can handle multiple asynchronous operations using
-
Q: What is a promise in JavaScript?
- A: A promise is an object that represents a value that will be available in the future or has already been computed. It can be in one of three states: pending, fulfilled, or rejected.
-
Q: Why is asynchronous programming important in JavaScript?
- A: Asynchronous programming is important in JavaScript because it allows multiple operations to be performed in parallel, keeping the application responsive and improving performance.
By mastering asynchronous JavaScript, you can significantly enhance the capabilities of your web applications and provide a better user experience.